http://blog.naver.com/techshare/100150127754
위에서 퍼온 글입니다.
정리해 보면, 결국 컴파일 된 DLL 이 Debug인지 Release인지는 Debuggable 이라는 특성에 지정된 값으로만 확인을 할 수 있습니다. 아래는 .NET Reflector 로 확인해 본 것입니다.
답이 모두 나왔군요. 즉, 해당 DLL 을 로드한 다음 assembly 레벨에 지정된 Debuggable 특성을 살펴보면 되는 것입니다. 그런데, 어떤 값을 봐야 하는 걸까요?
일단, Visual Studio 에서 Debug 모드로 빌드한 DLL 은 다음와 같은 특성을 포함하고 있었습니다.
반면, Release 모드로 빌드한 경우에는 결과가 다릅니다.
특성들을 보건데, 당연히 "DebuggableAttribute.DebuggingModes.DisableOptimizations" 옵션값이 중요합니다. 실제로, DebuggableAttribute 타입에 정의된 IsJITOptimizerDisabled 공용 속성도 다음과 같이 마이크로소프트에 의해서 코드 작성이 된 것을 볼 수 있습니다.
아래는, (DLL을 잠그지 않는) ReflectionOnly 버전으로 작성한 코드입니다.
위에서 퍼온 글입니다.
국내는 어떤지 모르겠지만, 해외의 경우에는 '서비스 중인 DLL'이 Debug 빌드된 것인지, Release 빌드된 것인지를 민감하게 여기는 경우가 있는 것 같습니다. 필요없이 성능에 손해되는 DLL 로 서비스할 이유는 없으니까요.
검색을 해보면, 생각보다 쉽게 방법을 찾을 수 있습니다.
How to tell if a .NET application was compiled in DEBUG or RELEASE mode? ; http://stackoverflow.com/questions/194616/how-to-tell-if-a-net-application-was-compiled-in-debug-or-release-mode
정리해 보면, 결국 컴파일 된 DLL 이 Debug인지 Release인지는 Debuggable 이라는 특성에 지정된 값으로만 확인을 할 수 있습니다. 아래는 .NET Reflector 로 확인해 본 것입니다.
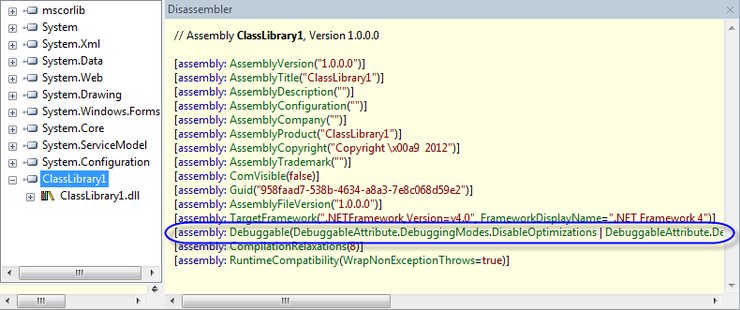
답이 모두 나왔군요. 즉, 해당 DLL 을 로드한 다음 assembly 레벨에 지정된 Debuggable 특성을 살펴보면 되는 것입니다. 그런데, 어떤 값을 봐야 하는 걸까요?
일단, Visual Studio 에서 Debug 모드로 빌드한 DLL 은 다음와 같은 특성을 포함하고 있었습니다.
[assembly: Debuggable(DebuggableAttribute.DebuggingModes.DisableOptimizations | DebuggableAttribute.DebuggingModes.EnableEditAndContinue | DebuggableAttribute.DebuggingModes.IgnoreSymbolStoreSequencePoints | DebuggableAttribute.DebuggingModes.Default)]
반면, Release 모드로 빌드한 경우에는 결과가 다릅니다.
[assembly: Debuggable(DebuggableAttribute.DebuggingModes.IgnoreSymbolStoreSequencePoints)]
특성들을 보건데, 당연히 "DebuggableAttribute.DebuggingModes.DisableOptimizations" 옵션값이 중요합니다. 실제로, DebuggableAttribute 타입에 정의된 IsJITOptimizerDisabled 공용 속성도 다음과 같이 마이크로소프트에 의해서 코드 작성이 된 것을 볼 수 있습니다.
public bool IsJITOptimizerDisabled
{
get
{
return ((this.m_debuggingModes & DebuggingModes.DisableOptimizations) != DebuggingModes.None);
}
}
아래는, (DLL을 잠그지 않는) ReflectionOnly 버전으로 작성한 코드입니다.
static void Main(string[] args) { Assembly asm = Assembly.ReflectionOnlyLoadFrom("ClassLibrary1.dll"); foreach (var attr in asm.GetCustomAttributesData()) { if (attr.Constructor.ReflectedType == typeof(DebuggableAttribute)) { foreach (var arg in attr.ConstructorArguments) { if (arg.ArgumentType == typeof(System.Diagnostics.DebuggableAttribute.DebuggingModes)) { System.Diagnostics.DebuggableAttribute.DebuggingModes mode = (System.Diagnostics.DebuggableAttribute.DebuggingModes)arg.Value; bool isDebug = mode.HasFlag(System.Diagnostics.DebuggableAttribute.DebuggingModes.DisableOptimizations); Console.WriteLine(asm.FullName + " ==> " + isDebug); } } } } }